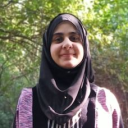
Hadia Saleem
We will begin with first understanding some basic concepts on intents and filters and then move on to implement them in our code.
In android, the data is shared through intents. The intent makes the sharing of any data easier and effortless. Now there are two ways an app can interact with the data, either the app can be used to share the data to an external app, for example sharing an image from Snapchat to WhatsApp, or to receive data for example storing a google image URL to a WhatsApp chat. The task in this post is to make the bubble.io app appear in the Share sheet so that a user can share an external link to the app and then store the shared data in the bubble.io app’s database.
In android, the data is shared in two ways. Initially, the data is shared between the two applications through ACTION_SEND. In this action, you define the data and its type. After the data is sent to the app, the intent resolvers immediately start the activity to handle the upcoming data.
The received data has a MIME type, the type can either be text, image, or video file, which is defined in the intent filter in the manifest file. It is a better practice to define each file type separately in <Intent filter> in <activity>. However, you can also set the type to “*/*” but in that case, you will have to make the intent handlers capable of handling any data type.
Now, there are three ways you can handle the received data in your bubble.io mobile application, an activity with a matching intent-filter tag, returning an object with a chooser target service, and sharing shortcuts published by your app. In this post, we will be using matching an intent filter tag with the activity, as it is more convenient. When an intent created by another application is passed to the startActivity( ), your app will appear on the share sheet. When the user will choose your application “MainActivity” will be started (as shown in the above code, second line).
After your application has received the data, it is up to you to define what to do with it, you can either store it in a variable, display it to the user, or in case it is a link, you can take the user to the shared link, etc. All of this can be done by getting the intent from the getIntent() function. When getting the intent make sure you have defined each data type and how to handle it as a user can send any data type.
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.intent.action.SEND" />
<category android:name="android.intent.category.DEFAULT" />
<data android:mimeType="*/*" />
</intent-filter>
</activity>
Copy
Step 2: Using the handleIntent() function
private void handleIntent() {
Intent intent = getIntent();
String action = intent.getAction();
type = intent.getType();
if (Intent.ACTION_SEND.equals(action) && type != null) {
if ("text/plain".equals(type)) {
handleText(intent);
}
}
}
Copy
The above code checks for the received intent type and then calls different functions based upon the data type.
Step 3: Handling the data type
void handleText(Intent intent) {
String sharedText = intent.getStringExtra(Intent.EXTRA_TEXT);
if (sharedText != null) {
my_url=sharedText.toString();
}
System.out.println("URL: "+ my_url);
}
Copy
When the code makes sure the data type is correct it calls the handleText function to store the shared URL in the my_url variable. Similarly, you can define what you want your program to do in the handleintent() function based on the received data or call another function based on the data type.
As shown below the shared link is stored in the my_url variable and to check if it works it is displayed using system.out.print command.
Also, check out our latest posts for more bubble.io tutorials. Happy bubbling!